Avatar
Avatar represents a person that contains an image or initials which can also be presented in a group with multiple avatars.
Introduction
The avatar component is usually seen for displaying user information in places such as menus, tables, and chats.
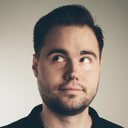
<Avatar />
Playground
Component
After installation, you can start building with this component using the following basic elements:
import Avatar from '@mui/joy/Avatar';
export default function MyApp() {
return <Avatar />;
}
Sizes
The avatar components comes with three sizes out of the box: sm
, md
(the default), and lg
.
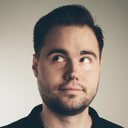
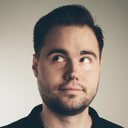
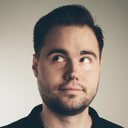
<Avatar alt="Remy Sharp" src="/static/images/avatar/1.jpg" size="sm" />
<Avatar alt="Remy Sharp" src="/static/images/avatar/1.jpg" size="md" />
<Avatar alt="Remy Sharp" src="/static/images/avatar/1.jpg" size="lg" />
Variants
The avatar component supports the four global variants: soft
(default), solid
, outlined
, and plain
.
<Avatar variant="soft" />
<Avatar variant="solid" />
<Avatar variant="outlined" />
<Avatar variant="plain" />
<Avatar>RE</Avatar>
Image
Insert images in the avatar by using a path inside the src
prop, similar to how you'd do in the HTML <img>
element.
Make sure to to write a meaningful description in the alt
prop.
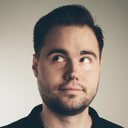
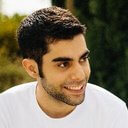
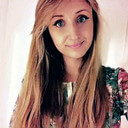
<Avatar alt="Remy Sharp" src="/static/images/avatar/1.jpg" />
<Avatar alt="Travis Howard" src="/static/images/avatar/2.jpg" />
<Avatar alt="Cindy Baker" src="/static/images/avatar/3.jpg" />
Image fallbacks
If an error occurs while loading the avatar image, it will fallback to alternatives in the following order:
- The provided children string.
- The first letter of the
alt
text. - A generic icon.



<Avatar alt="Remy Sharp" src="/broken-image.jpg">
B
</Avatar>
<Avatar alt="Remy Sharp" src="/broken-image.jpg" />
<Avatar src="/broken-image.jpg" />
With a badge
Combine the avatar component with the Badge
to extend what you can communicate with it.
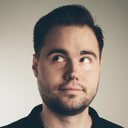
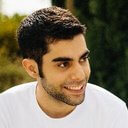
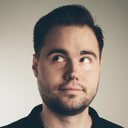
Group
Use AvatarGroup
component to group multiple avatars together.
import AvatarGroup from '@mui/joy/AvatarGroup';
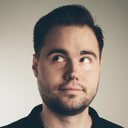
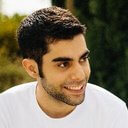
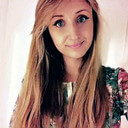
<AvatarGroup>
<Avatar alt="Remy Sharp" src="/static/images/avatar/1.jpg" />
<Avatar alt="Travis Howard" src="/static/images/avatar/2.jpg" />
<Avatar alt="Cindy Baker" src="/static/images/avatar/3.jpg" />
<Avatar>+3</Avatar>
</AvatarGroup>
Quantity within a group
The AvatarGroup
does not provide built-in props to control the maximum and the total number of avatars within a group.
This is because customization is broader if you have full control of the logic.
Use the snippet below as insipiration to create that:
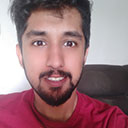
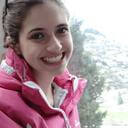
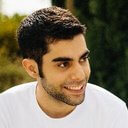
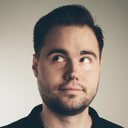
<AvatarGroup>
{avatars.map((avatar) => (
<Avatar key={avatar.alt} {...avatar} />
))}
{!!surplus && <Avatar>+{surplus}</Avatar>}
</AvatarGroup>
Ellipsis action
Avatar
exposes meaningful CSS variables to communicate with AvatarGroup
.
You can apply those variables to other components to mimic the avatar appearance inside a group.
This customization technique makes your interface more resilient to changes.
Here is an example of using IconButton
component to create an ellipsis action:
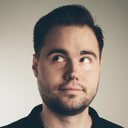
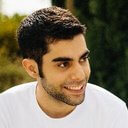
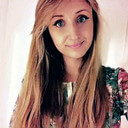
Overlapping order
By default, the first avatar in the group stays behind the second and so on.
You can reverse the overlapping order by reversing avatars position and using the CSS flexDirection: row-reverse
property in the AvatarGroup
.
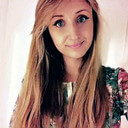
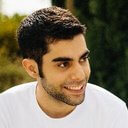
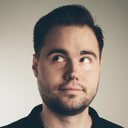
<AvatarGroup sx={{ flexDirection: 'row-reverse' }}>
<Avatar>+3</Avatar>
<Avatar alt="Cindy Baker" src="/static/images/avatar/3.jpg" />
<Avatar alt="Travis Howard" src="/static/images/avatar/2.jpg" />
<Avatar alt="Remy Sharp" src="/static/images/avatar/1.jpg" />
</AvatarGroup>
Vertical
To render the AvatarGroup
vertically, add the CSS writing-mode: vertical-rl
property to the it and rotate the extra element, if existent, by -90 degrees.
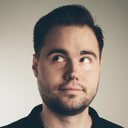
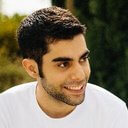
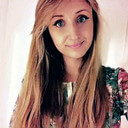
<AvatarGroup sx={{ writingMode: 'vertical-rl' }}>
<Avatar alt="Remy Sharp" src="/static/images/avatar/1.jpg" />
<Avatar alt="Travis Howard" src="/static/images/avatar/2.jpg" />
<Avatar alt="Cindy Baker" src="/static/images/avatar/3.jpg" />
<Avatar sx={{ transform: 'rotate(-90deg)' }}>+3</Avatar>
</AvatarGroup>
CSS variables
Play around with all the CSS variables available in the slider component to see how the design changes.
You can use those to customize the component on both the sx
prop and the theme.
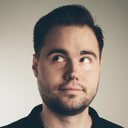
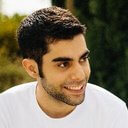
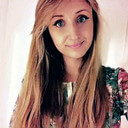
<AvatarGroup >
CSS Variables